What is the musical equivalent of Hello World? The C Major scale? A harmonized Happy Birthday? Pachelbel’s Canon?
When I wrote the above at 2 in the morning, I didn't expect to try to answer it seriously, but it's come across my mind a few times today, and I figured, if this blog is the place where I'm going to get all the junk out of my head, I might as well start here.
The "Hello, World" exercise is typically the simplest possible code that a programmer can write – literally, it just puts those two words on the screen, saying hello to the world. Still, it does serve a couple purposes. First, it lets the programmer know that something, anything, is happening – if nothing else, she at least knows that she can run her code successfully in her language of choice. Second, it's not the worst entry point to comparing unfamiliar programming languages. What it does is so basic that anyone can look at a piece of code and make some sort of judgment.
Even (or especially) if you've never written a line of code before in your life, you'd likely choose to start learning this language:
print "Hello, World!"
over this one:
.data
msg: .asciiz "Hello, World!"
.align 2
.text
.globl main
main:
la $a0,msg
li $v0,4
syscall
jr $ra
It's important to note that the former, in Python, has a lot going on under the hood. There's a lot happening that the programmer isn't necessarily aware of – it may come back to bite her in the future when she tries to do something more complicated, or it may not. The latter example, in assembly, looks much more like "code" than English, but it gives the author finer-grained control over exactly what happens.
I'd have to think that for Western Classical music, the equivalent to a "Hello, World" program is either playing a scale or a note. Playing a scale is the first thing we're often taught when we first approach an instrument, but I think there's plenty to unpack just in playing a single note.
Comparing instruments to programming languages, playing a note on the piano is like writing "Hello, World" in Python (print "Hello, World"
). It's possible for someone to literally let their hand fall and let gravity play the note for them, ignoring the complexity under the hood (or in this case, the piano lid).
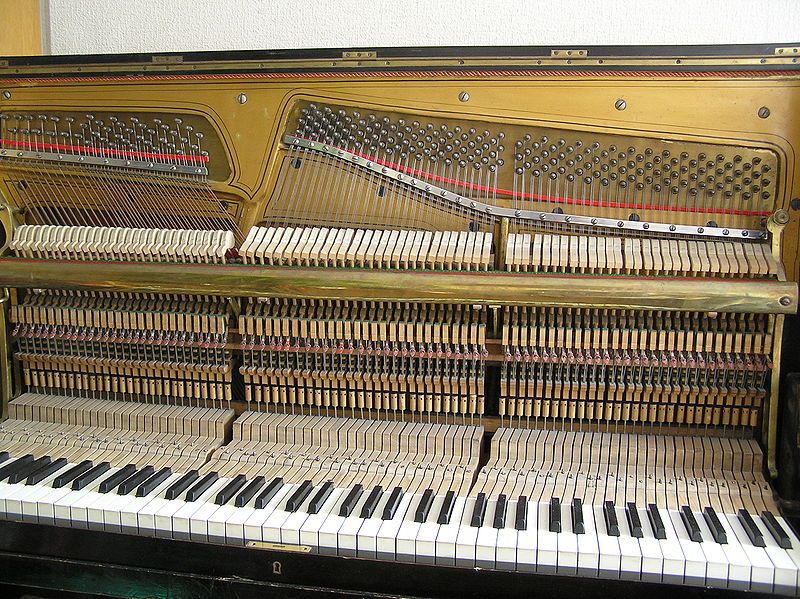
On the other hand, a string instrument (i.e. violin, viola, cello, bass) requires the proper contact between bow and string, a properly tuned instrument, a rosined and tightened bow, placement of the bow at the right angle at the right part of the instrument, and a steady pull of the bow. In return, the player gets much more control over the sound than they would on a piano, including the ability to make a note get louder after playing it and my personal source of jealousy, the ability to vibrate the pitch. Here's a violinist's take:
This trade off of low-level effort for a great deal of control resembles the C programming language quite a bit.
#include <stdio.h>
int main() {
printf("Hello, World!\n");
return 0;
}
I've never studied the woodwinds or brass, except for the one time I tried to make a sound on a friend's flute and failed miserably. Because you blow across the blowhole, not into it, getting the air flow right feels to me like magic. As for a programming language, how about Java, whose incantation is so magic-filled that it will often take a new student months before they really understand everything that's happening?
class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
It's important to say that once you get past "Hello, World" to writing a non-trivial piece of code, everything changes. Likewise with playing an instrument.
I'd like to go back now to the Python of instruments, the piano, and what its "Hello, World" might look like in more detail. Not too long ago Professor Robin McCabe asked me what verb I might use to describe how you physically make sound on the piano to someone on the street. My cello teacher growing up, the wonderful Lois Errante, had a way with these kinds of explanations; one in particular stays with me still: "Let the left hand fingers hang like off a window ledge." Alas, one doesn't play piano by hanging off the keys from below, Glenn Gould notwithstanding. I knew what the naive-but-wrong answer was – you don't "press" the keys since you'd end up a tight mess with your fingers and arms locked up, unable to produce much nuance – but the right answer escaped me.
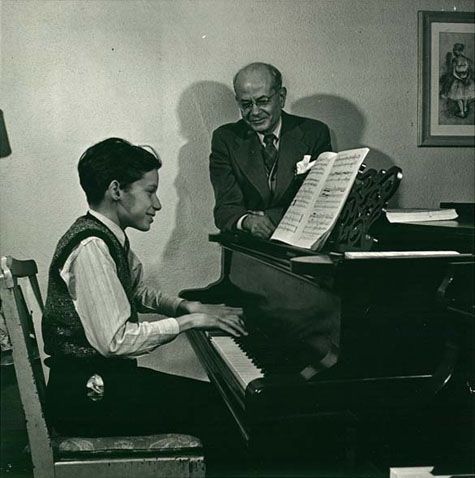
Before my long time piano teacher, Natalie Maynard, passed away, she gave me photocopies of a dozen or so pages of her notes from Juilliard which startled me then, and which to this day I look back on from time to time. They're from her time with Sascha Gorodnitzki, one of the great piano pedagogues of the day, with whom she studied towards the end of a seven-year graduate scholarship.
When Natalie gave me these notes, she did so with no introduction. I was in high school at that point, and she merely mentioned that they may interest me in some capacity. I went home after that lesson, eager to get a glimpse at what advanced secrets she might have been privy to at that point in her education, and I set to work deciphering her scribblings.
They begin:
I. Scales
- Rest hand without sounding on 1st 5 notes of C Maj.
- Turn hand slightly (out) so that thumb lies at an angle on key (weight of hand leaning towards thumb)
- depress thumb (from surface of key - not alone - this will prevent a bang thumb) + simultaneously raise curved 2nd finger to height of knuckles (keep 2nd finger curved - never straight - just from big knuckle)
etc.
She was studying how to play a C Major scale! Often the very first exercise given to a brand new piano student of any age (and if you recall, one of my candidates for a musical "Hello, World"), she was going over it at the end of a graduate degree at Juilliard – and it's not because she never learned it. There are any number of lessons to be extracted from this – the importance of fundamentals being chief among them – both from the fact that Gorodnitzki was compelled to teach the C Major scale at this stage and the fact that Natalie was compelled to take notes about it.
Then there is the question of the content of these instructions, at once both obvious and specific. Many pianists, upon reading them, will try them on for size, mimic the steps on a table, think "that looks about right" and move on. Others will find points of disagreement, perhaps in the details of step 3. Others still will think, "I wish this was explained more like 'hang from the window ledge'. That's much easier to follow."
But in this particular instance, I'm still looking for a verb to describe how a pianist makes sound on the instrument. There's "rest", "turn", "depress", and "raise". Taken together (modulo some notion of horizontal motion), these actually do tell most of the story of playing the piano – "depress" being the one that is closest to the actual production of sound. I'm no linguist, but my hunch is that "depress" really isn't that far off etymologically from "press." Still, the connotation is different. If instead of telling a student to "press" the keys, you instruct him to "depress" them, you may occasionally end up with some dark minor-key music, but most of the time the student will end up with less tension at the instrument.
What less tension really translates to – whether or not it results in a better or even different tone being produced from the instrument – is beyond the scope of this first post. The piano is a marvelously complex instrument, and there's much to understand past print "Hello, World"
. I hope to explore a bit of this in the writing to follow.
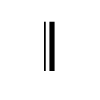